Signals Slots Qt Core 6.7.3
Signals & Slots
GUI programming often wants to notify other widgets when changing a widget. More in general, we want to be able to communicate with all kinds of objects. For example, when a user is pressed, a window Close () function is called.
In other toolkits, this bond is implemented by callback support. Callback is a pointer to a function, so if you want to notify the handler function an event, pass a pointer to another function (callback) to the handler function. The handler function calls a callback when necessary. However, some frameworks are successful using this method. Callback is likely to be intuitive, and it is likely that it will be difficult to check whether the callback argument is correct.
Signals and Slots
QT uses signals and slots as candidates for callback techniques. The signal is emitted when a specific action occurs. There are many defined signals in QT widgets, but you can subdivide widgets and add their own signals. The connector is a function called in response to a specific character. QT widgets have a large number of defined slots, but it is common to add a custom slot in a subclass, so you can customize the signals you are interested in.
The mechanism of the signal and slot is type safety: Signal signature must match the signature of the receiving slot. (In fact, the connector has a function to avoid unnecessary arguments, so it can have a signature shorter than the received signature). Since the signature is compatible, when using a function pointe r-based syntax, the compiler is useful for detecting type mismatch. Using the rule s-based signal syntax and Slot syntax, you can detect type mismatch while running the program. The signal and slots are gentle connected, and the class that emits the signal does not understand or care which slot receives the signal. In the QT signal and the slot structure, it is guaranteed that combining signals and slots will be called when a connector with signal parameters is required. Signal and slot can take any number of arguments. They are completely harmless to species.
Every class that inherits from QObject or its subclasses (such as QWidget) can contain signals and slots. Signals are emitted when an object changes its state in a way that might interest other objects. This is the only communication an object makes. An object does not know or care if anything receives the signals it emits. This is a true encapsulation of information that allows objects to be used as software components.
Slots can also be used to receive signals, but they are also ordinary member functions. Just as an object does not know if it is receiving a signal, a slot does not know if it is receiving a signal. This allows Qt to create truly independent components.
A slot can have as many signals connected to it as you want, and a signal can have as many slots connected to it as you want. A signal can also be directly connected to another signal (in which case the second signal is sent immediately after the first signal is sent).
The combination of signals and slots allows a powerful component programming mechanism.
Signals
Signals are emitted by an object when its internal state changes in some way that might be of interest to a client or owner of that object. Signals are public functions and can be emitted from anywhere, but it is recommended that they be emitted only from the class that defined them and its subclasses.
When a signal is emitted, the associated slot is usually executed immediately, like a normal function call. In this case, the signal and slot mechanism is completely independent of the GUI event loop. The code following the emit instruction is executed after all slots have returned. In this case, the code following the emit keyword is executed immediately, and the slots are executed later.
If multiple slots are connected to a signal, when the signal is emitted, the slots are executed one after the other in the order they were connected.
Signals are generated automatically by the moc, so they do not need to be implemented in the . cpp file.
Note about arguments: in our experience, signals and slots are more reusable if they do not use special types. If QScrollBar::valueChanged() used a special type like QScrollBar::Range, it could only be connected to slots designed specifically for QScrollBar. It would not be possible to connect different input widgets.
Slots
Connectors are called when a signal is connected. Slots are considered as normal C++ functions and are quite likely to be called in a straightforward way.
Because slots are considered as normal member functions, they follow simple C++ rules when called directly. However, because they are slots, they may be triggered by at least one component, autonomously from the access value, by signal-slot fusion. This means that a board issued by an instance of any class may call a personal connector in an instance of a non-related class.
Slots can also be qualified as virtual slots.
Compared to current calls, signals and slots run slightly slower, since they provide greater resiliency, but in real-world applications the difference is not significant. In general, a signal signal associated with several slots will be about 10 times slower than a direct call from a receiver that supports non-recent feature challenges. These are the costly costs required for searching for objects of connections, for conducting non-use of all connections (i. e. testing that further receivers were not destroyed during the emission of the signal), and for marching through each feature in the overall look. However, 10 issues of harmless functions have every chance of rising in number, and these are significantly cheaper costs than any operation of, say, new or delete. If you perform an operation on a row, vector or list that is inadvertently called new or delete behind the scenes, the cost of the signal and slot is only a fairly small part of the total cost of calling the function. The same is true if you make a system call inside a slot, which can indirectly call 10 or more functions. This cost is incurred because the signal and lock mechanism is simple and resilient.
Beware that other libraries that define variables called signals or slots may cause warnings or compilation errors during compilation near your QT-based application. To solve this problem, set the preprocessor character to #UNDEF.
A Small Example
A minimal class in C++ has the following features:
classCounter< public: bar()< m_value = 0; > subscribemeaning()const < return m_value; > deletereturn setValue(subscribevalue);private: subscribem_value; & amp; amp; gt ;;
A small class based on QOBject has a habitual look:
#includeclassdeskpublic Qobject < Q_OBJECT // Attention. The macro Q_Object starts the private section. // To announce public members, use the access modifier 'Public:'. public: bar()< m_value = 0; > subscribemeaning()const < return m_value; > public slot: deletereturn setValue(subscribevalue);signal: deletePrice change (subscribeNEWVALUE)private: subscribem_value; & amp; amp; gt ;;
The QOBJEC T-based version has the same internal state and provides public methods to access it, but also supports component programming with signals and slots. This class has a slot that allows you to transmit your condition to the outside by sending e-mail to the ValueChanged () signal, and can send a signal for other objects.
All classes, including signals and slots, must be described as Q_object at the top of the ad. It must also be derived from QOBJECT (directly or indirectly).
The slot is implemented by the applied programmer. The following is an implementation example of the counter slot :: setValue ():
deletecounter::setValue ()subscribevalue)< if(meaning!=m_value)< m_value =Does meaning;Return the messageValueechanged (value); & amp; amp; gt; & amp; gt;
EMI T-Round sends the ValueEechanged () signal from the object with a new value as an argument.
The following code creates two counter objects and connects the first object ValueChangeded () signal and the second object setValue () slot () slot () slot ().
To,B;Qobject::connect(&a, &counter::Price change, &b, &counter::Set value); A.setValue ()12); // A. value () == 12, B. value () == 12 b.setValue ()48); // A. value () == 12, B. value () == 48
A. SetValue Call (12) sends a signal ValueEchanged (12) to A, and B receives it with slot SetValue (). Next, B sends the same ValueEchanged () signal, but the signal is ignored because the slot is not connected to the Signal B 'ValueChanged ().
Function SetValue () is Value! Please note that the value is set and the signal is output only when = m_Value. This can prevent endless bins for periodic connections (for example, if B. ValueChanged () is connected to A. SetValue ()).
By default, one signal is issued for each connection, and two signals are issued for duplicate connections. Call Disconnect () once to disconnect all these connections. When the Qt :: UniqueConnction type is transmitted, the connection is established only when it is not duplicated. If there is already an overlap (exactly the same slot of the same object), the connection will not succeed and will connect the fake.
This example indicates that objects can work together without knowing all information about each other. To do so, you only need to connect the object between each other, and use a simple call of the QOBJECT :: Connect () function or the UIC automatic connection function.
A Real Example
Below is a sample title of a simple widget class with no member functions. The purpose of this class is to show how to use signals and slots in an application.
LCDNumber inherits from QOBject, which has most of its knowledge about signals and slots through QFrame and Qwidget. It is somewhat similar to the built-in qlcdNumbe r-widget.
The macro Q_Object extends the preprocessor to declare various member functions that are implemented by MOC. If you get a compiler error like "undefined reference to VTable for LCDNumber ", you probably forgot to start MOC or to record the MOC output in the link team.
public: Lcdnumber (Qwidget *parent=nullptr);signal: deleteoverflow();
Following the class designer and open members, declare the signals for your class. The LCDNumber class emits a signal called overflow() when it is asked to display something that is not possible.
If you don't care about overflow, or you know that overflow won't happen, you can ignore the overflow() signal.
On the other hand, if you want to call two different functions on error when a number overflows, just connect the signal to two different slots; QT will trigger both (in the order they're connected).
public slot: deletedisplay(subscribenum);deletedisplay(doublenum);deletedisplay(const Qstring &str);deletesethaxMode ();deletesetDecMode ();deletesetoctMode ();deletesetBinMode();deletesetsmallDecimalPoint();bootPOINT); & amp; amp; gt ;;#Endif
Slots are receiving functions used to get information about changes in the status of other widgets. The LCDNumber shown in the code above uses this to install the number being displayed. The slot is public because Display() is part of the class interface to other programs.
In some example programs, the ValueEchanged() QScrolbar signal is connected to the Display() slot so that the LCD-price always displays the scroll strip.
Note that Display() is an overload. When you connect a signal to a slot, QT will choose the corresponding version. For the reverse challenge, it has to look for five different names and check the type of each independently.
Signals And Slots With Default Arguments
Signals Signals and slots can have arguments, and arguments can have default values. Consider qobject :: destroyed ():
deleteWhen QOBJECT is deleted, a signal called QObject :: Destroyed () is issued. If there is a link to the external QOBJECT, I would like to intercept this signal and delete it. The appropriate signature of the lock is as follows:Qobject* =ObjectDestroyed (
QOBJECT
deletenullptr);Qobject*Connect (sender=ObjectDestroyed (
Broken
this, &Qobject::ObjectDestroyed);, There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit., &You can also connect to C ++ 11:::Connect (Sender)
QOBJECT
Destroyed
this, &Qobject::ObjectDestroyed);, There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit., [=]()< There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit.->When the sender or context is discarded, Lambda is disconnected. When the signal occurs, it is necessary to make sure that all objects used in the function are alive..Another way to connect the signal and the slot is to use QOBJECT :: Connect (), Signal Macro and Slot Macro. If the argument has a default value, the rule of whether or not to include or not in the macro of Signal () and Slot () is the signature sent by the macro () macro than the signature sent by macr o-slot (). Don't have a small argument.
All of these options work:
Connection (sender
signal(
QOBJECT
this,Lock (object destruction (QBJECT))Qobject*)), There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit.,QOBJECT*this,Lock (object destruction (QBJECT))Qobject*)), There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit.,Lock (ObjectDestroyed ()));,But this option does not work:, There are several advantages of using QOBJECT :: Connect () and functio n-indicators. First, the compiler can check if the signal argument is compatible with the argument of the slot. If necessary, the compiler can also convert arguments in tacit.,Note that when using this overload QOBJECT :: Connect (), the signal and slot arguments are not checked by the compiler.
If you need information about the signal sender, Qt provides a QOBJECT :: Sender () function.
Lambda type is a convenient way to transfer user arguments to slots:
Connect (Action)
Advanced Signals and Slots Usage
QACTION
Movement
engine, &Matrix (action::Text ()); & amp; amp; gt;);,QT and manufacturer's signal / lock can be used together. You can also apply both mechanisms with the same plan. To do this, make an appropriate description in the CMAKE planning file:, [=]() < engine->Target_compile_definitions (My_pp Private Qt_no_keyWords).->The Qmake (. Pro) plan should be compiled:
Using Qt with 3rd Party Signals and Slots
Configuration
NO_KEYWORDS
This indicates that QT has not defined MOC's main text (signal, slot, emanication) in advance. In order to continue introducing Q t-Signals and Slots with the No_keywords flag, the first text of all Qt Moc's main text, Qt Q_Signals (Q_SIGNAL), Q_slots (or Q_slot), Q_EMIT Please change it.
In exchange for the signal and the slot, apply the main text Q_Signals and Q_slots of the published Q T-based API library. Unfortunately, this library is difficult to apply in a plan where Qt_no_keywords is defined.+=In order to guarantee this limit, Library developers can introduce the definition of processor QT_NO_SIGNALS_SLOTS_KEYWORDS when compiling libraries.
This definition eliminates signals and slots, and does not trade all the possibilities of other Q T-specific main texts used in the implementation of the library.
Signals and slots in Qt-based libraries
В© 2024 The Qt Company LTD. documentation is considered a copyright driver certificate from the appropriate owner. The document submitted in the actual document has been licensed under the GNU Free Documentation Lice Criteria published by Free Software Foundation. The QT and the right logo are considered a product symbol of QT Company LTD. in Finland and / or other countries. It is regarded as a product symbol. All other trademarks are regarded as the property of the right holder.
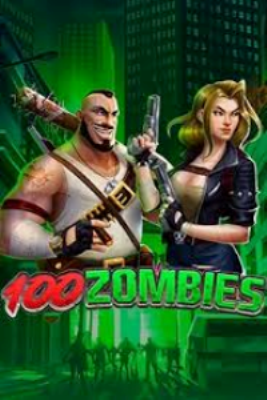